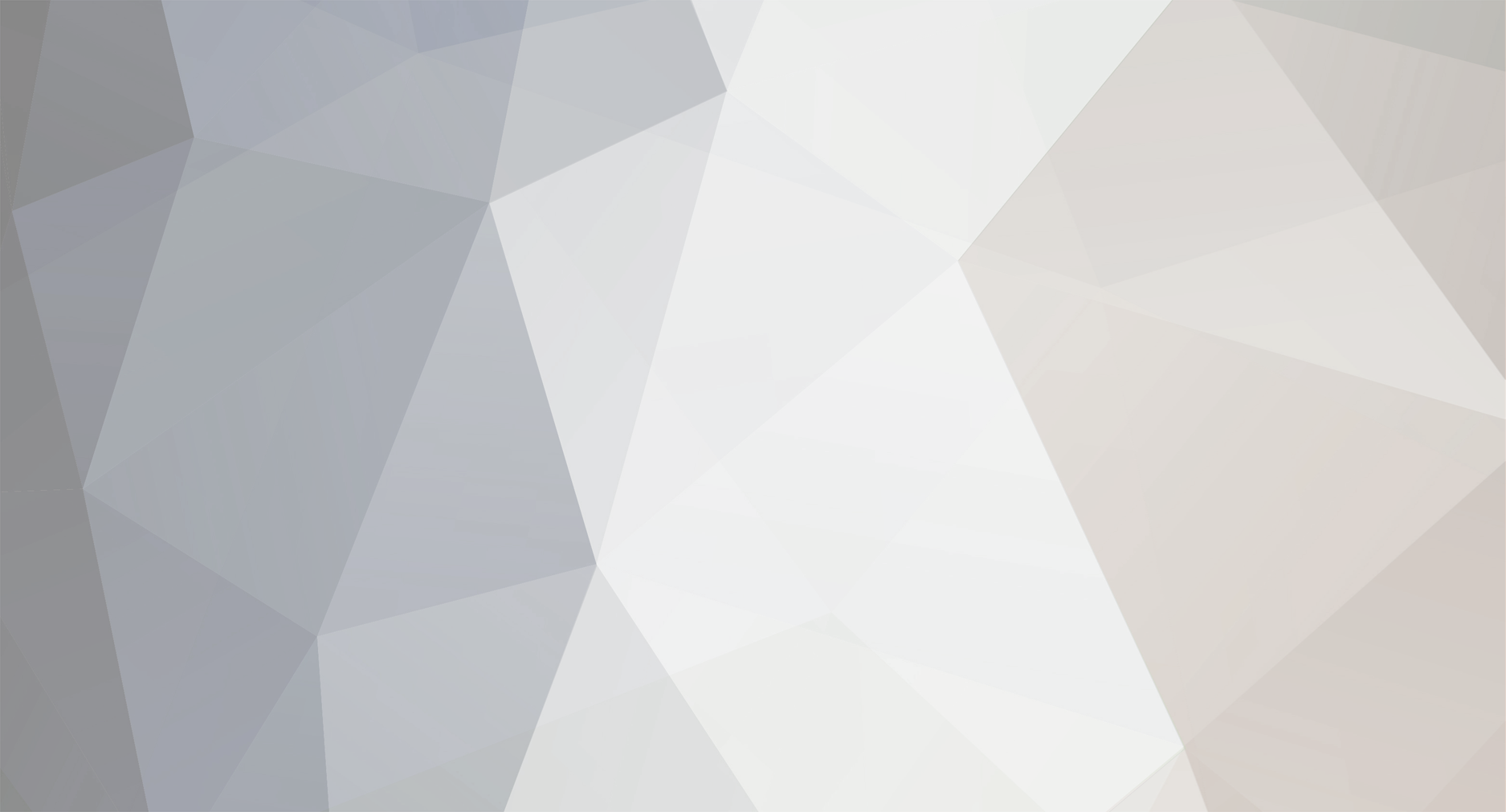
Cudabear
Members-
Posts
87 -
Joined
-
Last visited
Everything posted by Cudabear
-
Hi all, Just wanted to share a workaround I made today while trying to increase the performance of tilemaps in my game. My game has maps that could be 2048px X 2048px (64x64 32px X 32px tiles) or maybe even bigger down the road, and contain up to 8 layers for applying detail. The problem I ran into was, when scrolling the camera, I was seeing huge performance drops, even on powerful computers. Turns out, Phaser was redrawing the tilemap every frame you move the camera. My tilemap, after being loaded, never really changes. So I wanted to find an easy way to force Phaser to draw the entire tilemap once and then use it for a static sprite after that. I tried using https://photonstorm.github.io/phaser-ce/Phaser.TilemapLayer.html#cacheAsBitmap, but it didn't seem to work. Not sure if I was missing something there. So my solution was to resize the layer before getting the texture: myLayer.resize(game.world.width, game.world.height); myLayerTexture = myLayer.generateTexture(1, PIXI.scaleModes.DEFAULT, game.renderer); myLayer.visible = false; game.add.sprite(0, 0, myLayerTexture); this way, instead of the tilemap being added as a dynamic thing that gets redrawn constantly, it functions as a static image. But because each layer is still treated as a separate image, I preserve rendering order. In the background, I keep the actual layers hidden to preserve collision data. Unfortunately, if the tilemap were to change ever beyond initial creation, the texture would need to be updated, which would be expensive. But since I'm not really planning on doing that, it seemed like a reasonable solution to me. Hope this helps someone else who has this problem.
-
Hard to tell without seeing the rest of code. What you've posted seems like it should be working. Have you enabled arcade physics on PlayerHitbox, Player, and Enemy1BGroup?
-
Looks like you'll be on your own for this one. You could build it using some logic and well-positioned sprites, but that would take a lot of work. Since you have full access to the DOM anyway, you may just want to float a select over your canvas at the correct position, and then use CSS to style it as close as possible to match your game. It should save you a lot of time in the long run.
-
So your line: diamond.reset(350, 350) sets the diamonds to spawn at that exact position in the world each time you make a new one (x350, y350) You can use https://photonstorm.github.io/phaser-ce/Phaser.World.html#randomX and https://photonstorm.github.io/phaser-ce/Phaser.World.html#randomY to get a random position in the world to spawn them in. as such: diamond.reset(game.world.randomX, game.world.randomY) This may spawn the diamond inside the tilemap, so you may want to work around that somehow.
-
For the first one, you're calling it every time through the update loop. You can use justPressed to prevent this https://photonstorm.github.io/phaser-ce/Phaser.Key.html#justPressed. if(controls.attack.isDown){ player.body.velocity.x = 0; if(controls.attack.justPressed) { //only play the animation if the user just pressed the key player.animations.play('attack', 7, false); } } For the second one, your idle animation is getting played every time your player sprite doesn't have x velocity. Since the jump is called whenever the user is jumping, I'd do something like this: if(!controls.attack.isDown){ if(player.body.touching.down){ //if we're on the ground, handle ground animations if(player.body.velocity.x === 0){ player.animations.play('idle'); } else { //walking animations???? } } else { //if we're in the air, we're obviously jumping or whatever player.animations.play('jumping'); } }
-
Check if my sprite has been overlapped by any sprites in this group
Cudabear replied to My Evil Pony's topic in Phaser 2
You can pass a group as the arcade physics overlap function. https://photonstorm.github.io/phaser-ce/Phaser.Physics.Arcade.html#overlap That said, you need arcade physics on all sprites within the group as well as the sprite you want to check against the group. If you feel you don't need physics on the group's sprites, check this example: https://www.phaser.io/examples/v2/sprites/overlap-without-physics Also, for future reference, you can forEach any group do custom work on each of its elements independently, just like a vanilla javascript array. -
I don't believe Phaser does anything other than importing the tileset image. It might be a browser specific issue with the way you exported your tileset. I'd play around with different browsers and image file formats to see if you can fix it. That said, I've never seen this issue with png tileset images in chrome.
-
You could probably set the character sprite to be a child of the ball, with the appropriate offset of the default state (let's say moving to the left). Then, when the player moves to the other state (the right) flip the x scale of the character sprite, from 1 to -1. The character should now be facing the opposite direction on the other side of the ball.
-
I'm pretty sure body.touching never cares to check to see what it's touching. In order to do this, you'll probably want to add all the sprites that you want to affect by touching to a group, then call game.physics.arcade.collide(sprite, group, function(sprite, collidedSpriteFromGroup) { //affect collidedSpriteFromGroup here }); inside your update loop. This example uses overlap instead of collide, but it's effectively the same thing: http://phaser.io/examples/v2/arcade-physics/custom-sprite-vs-group
-
Tip: use non-minified Phaser for dev to get more descriptive errors. Looks like your animations are failing to play. Have a look at player.animations.add('left', [0, 1, 2, 3], 10, true); player.animations.add('right', [5, 6, 7, 8], 10, true); And see if those indexes are configured correctly (you have enough frames in the image, and so forth.
-
As far as I know, this isn't really feasible because there's no way to know how big the file being downloaded is. It's possible to get the `header` information from the request to get the total number of bytes downloaded already, and then compare that to some hard-coded value about the size of the value (or fetch the size of the file via some back-end request) but that seems fragile and too heavy-handed. Most Phaser games show progress as a percent of total files downloaded (not great if you've got a big sprite atlas, or large music files, but it's something at least) Here's my example of how I show progress of loaded files: https://github.com/Cudabear/ldwrap/blob/master/src/state/LoadState.js The important line is `this.load.setPreloadSprite`.
-
I linked the github in the post. It was made for a gamejam so it's all open source. Feel free to take, use, and refactor anything.
-
Awesome! Good work on your game too It would be a shame for you not to post it just because you missed the Compo deadline!
-
@MikeW You can still submit for the Jam, for about 8 more hours.
-
Yeah! We managed to get just about everything we wanted to get into our game: http://www.cudascubby.com/ld38 It's mostly content heavy, involved a lot of scripting and building systems to allow for scripting, and was an interesting experience. We created 10 unique rooms, 3 unique enemy types, a mini boss, a boss (sort of), and 2 endings. It should take less than 10 minutes to beat. You can find the source here as well: https://github.com/Cudabear/ld38
-
I'd assume it would be fine, provided there's no naming conflicts. Depending what you want to do, you could probably just instantiate a phaser game object inside a controller or directive. If you need this to persist beyond the scope of that controller/directive, maybe you could potentially put it in a service. You could even put it in a RunBlock, and it would be fine. I guess it all depends on what you're trying to do with Angular on top of Phaser.
-
Here's a good way to do it using two rectangles: https://phaser.io/examples/v2/geometry/rectangle-intersects You could just use the bounds of the sprite instead of one of the rectangles, then check if intersects exists and has height/width to determine if a part of the sprite is within the target rectangle. Furthermore, you could check to see if intersects has the same bounds as your sprite if you want to see if the sprite is all the way inside the rectangle.
-
What I typically do is have a game state that corresponds to your main gameplay loop, IE when your user is actually playing the game. Then, I feed this gameplay state a data object that represents the level that the player will be playing on, with information such as where the character spawns, where the goal object(s) spawn, where enemies spawn, gameplay triggers, ect. The main loop can parse out this data and actually calculate the logic on the fly. This way, you create degrees of separation between your gameplay logic and the data that represents your "worlds" and it'll be much easier to add more worlds or change mechanics later. Then, when it comes time to switch to a new world, you just have to restart the state again with a new "world" data object.
-
Long time Phaser Ludum-Darer here! Going to be my 8th time entering a Phaser game, crazy! Good luck on your game. We'll have to use this thread later to share our creations.
-
I personally ran into this problem when I first started learning Phaser and I've helped dozens of people through it as well over the years, so it leads me to think there's a gap in the documentation there. However, the examples do clearly show you need to use velocity, so who knows. I think it's just an easy hole to fall in when first using Phaser, when you start with moving positionally and then try to throw in collision you naturally think your positional moving logic will just work. If more tutorials skipped positional moving and just did stuff with velocity/tweens it would probably be less of an issue, but you never know.
-
A common mistake! Your problem is here: if (player.cursor.left.isDown) { player.image.position.x -= 10; } else if (player.cursor.right.isDown) { player.image.position.x += 10; } else if (player.cursor.up.isDown) { player.image.position.y -= 10; } else if (player.cursor.down.isDown) { player.image.position.y += 10; } } When working with physics, you can't directly move sprites around with their positional values and expect them to collide with other sprites. The reason for this is physics calculations require velocity, and if you directly move things around the velocity of these objects is 0, so no calculation can be done. Change your code to something like this if (player.cursor.left.isDown) { player.image.body.velocity.x = 10; } else if (player.cursor.right.isDown) { player.image.body.velocity.x = 10; } else { player.image.body.velocity.x = 0; } if (player.cursor.up.isDown) { player.image.body.velocity.y = 10; } else if (player.cursor.down.isDown) { player.image.body.velocity.y = 10; } else { player.image.body.velocity.y = 0; } And the collisions should work. Also, position calculated via velocity is weird and might not be the same as moving sprites directly, so you might need to fidgit with the number 10 to something that feels better for your game. Good luck!
-
I'm not entirely sure you can't, but I'd really suspect that you can't. Those parameters that you pass in (row, column) essentially get directly put as indexes of the double-indexed array that represents the map. Arrays by definition can't have things at negative indices, so there's your answer. The requirement of having a map that grows in all directions is a tough one. You could make the leftmost column 0 and the topmost row 0, and then when the player moves outside of those bounds, you could prepend the new tiles onto the front of the row and column arrays, so you maintain that the topmost and leftmost tiles are index 0. But that might be computationally expensive. Also, because tilemaps must be squares or rectangles, you might have a massive tilemap that represents what's essentially long strips of tiles when players wander off in one direction. In the end, you're going to run in to scalability problems where your server is sending massive amounts of JSON data to your clients to update them on the state of the world. How games that have "infinitely expanding worlds" usually get around this is to have "chunks", or similarly smaller-sized pieces that fit together to look seamless. What I would do here is make your chunks individual tilemaps (of small sets of tiles, maybe 50x50) that are indexed on the server as a double-indexed array. Have the client then request the required number of chunks around them from the server, and load them in to make the game look seamless (using offsets on the individual maps to align them in the world, even though they're technically all have their own 0 indexes in the top left). When the client requests a chunk from the server that hasn't been generated yet, have the server generate a new tilemap for that chunk and send it out, then cache it for other clients to see when they visit the same area. In theory, your server will then need to store this double-indexed array of tilemap JSON files in some kind of database. You'll also need to do some experimentation with how the JSON from tiled looks and replicate it if you want the server to generate it. I hope this helps. Let me know if you have any questions.
- 3 replies
-
- coordinates
- tile
-
(and 1 more)
Tagged with: