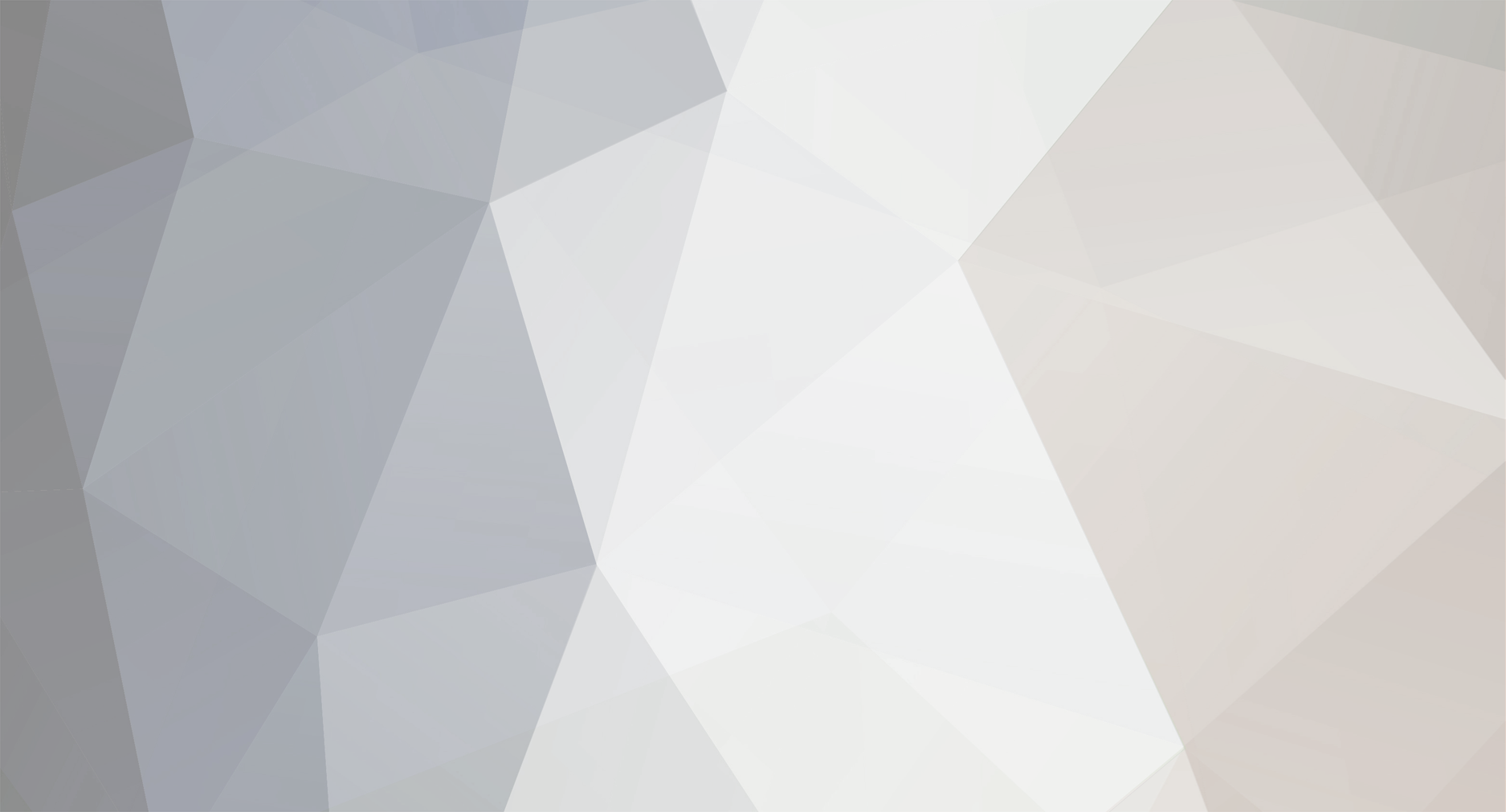
AndrewJS
Members-
Posts
11 -
Joined
-
Last visited
Everything posted by AndrewJS
-
Yeah I still use the Typescript namespaces, haven't switched to import/export yet. I have a big framework that would take a while to port to ES6. I can see some advantages of import/export for things like multiple definitions but it also ties the imports to the file paths so moving/renaming things can get messy with a lot of files. For the namespaces, I was looking at PIXI.spine but it doesn't seem to have all the definitions. When I log that in the console from the UMD file, it produces: PIXI.spine; AttachmentType: {0: "Region", 1: "BoundingBox", 2: "Mesh", 3: "LinkedMesh", 4: "Path", 5: "Point", 6: "Clipping", Region: 0, BoundingBox: 1, Mesh: 2, LinkedMesh: 3, Path: 4, …} BinaryInput: class BinaryInput Color: class Color DebugUtils: class DebugUtils IntSet: class IntSet Interpolation: class Interpolation MathUtils: class MathUtils Pool: class Pool Pow: class Pow PowOut: class PowOut Spine: class Spine$3 SpineBase: class SpineBase SpineMesh: class SpineMesh SpineParser: class SpineParser SpineSprite: class SpineSprite StringSet: class StringSet TextureAtlas: class TextureAtlas TextureAtlasPage: class TextureAtlasPage TextureAtlasRegion: class TextureAtlasRegion TextureFilter: {9728: "Nearest", 9729: "Linear", 9984: "MipMapNearestNearest", 9985: "MipMapLinearNearest", 9986: "MipMapNearestLinear", 9987: "MipMapLinearLinear", Nearest: 9728, Linear: 9729, MipMap: 9987, MipMapNearestNearest: 9984, MipMapLinearNearest: 9985, …} TextureRegion: class TextureRegion TextureWrap: {10497: "Repeat", 33071: "ClampToEdge", 33648: "MirroredRepeat", MirroredRepeat: 33648, ClampToEdge: 33071, Repeat: 10497} TimeKeeper: class TimeKeeper Utils: class Utils Vector2: class Vector2 WindowedMean: class WindowedMean filterFromString: ƒ filterFromString(text) settings: {yDown: true, FAIL_ON_NON_EXISTING_SKIN: false, GLOBAL_AUTO_UPDATE: true, GLOBAL_DELAY_LIMIT: 0} wrapFromString: ƒ wrapFromString(text) I can't find classes like AnimationStateData or anything from the runtime folders. These used to be in PIXI.spine.core but these files are now in the runtime-4 folder and I don't know which namespace they are placed in the UMD output. Once I can get it working I'll post the result. I have done something similar for ThreeJS and it worked out fine, just had to rename some things manually.
-
The Spine runtime for Pixi supports Spine version 4 now but the project transitioned to ES6: https://github.com/pixijs/spine It outputs an ES5/UMD build for the Javascript but without Typescript definitions. The 3.8 version had these definitions. "Vanilla JS, UMD build All pixiJS v6 plugins has special umd build suited for vanilla. Navigate pixi-spine npm package, take dist/pixi-spine.umd.js file. Unfortunately, there are no typescript definitions for vanilla build on both pixi v6 and pixi-spine" I can get the class definitions from the ES6 output but I don't know the namespaces used in the UMD version. How are the namespaces assigned to each class when generating the ES5 output? What would be the easiest way to produce Typescript definitions for the UMD version? I guess the same applies to Pixi 6 but I don't need to upgrade to this until later.
-
Great, thanks for the reply. I'll have a look at the compressed texture module. https://github.com/pixijs/pixi-compressed-textures
-
When an image loads in Pixi, I assume it loads into memory as an HTML object and is copied into a WebGL (or other) texture buffer, meaning it uses 2x the memory of the image itself? A 2k image texture for example is 2048 x 2048 x 32-bit = 16MB. In a WebGL app, would this be 32MB of memory plus overhead? If so, is there a way to get Pixi to flush the source images from memory and just keep the WebGL buffers or does it need to retain the source images, for example, to generate buffers in different WebGL contexts?
-
If I render blend modes like add or multiply in a render texture, they render with black where the transparency would be if I render them against a background. I don't think I can get the blend mode to affect background layers when rendering into a render texture, I'd probably have to put each layer with the same blend mode in a separate render texture and use the blend mode on the whole texture. Is there a way to have the blend mode layers render transparent instead of black in the render texture though?
-
I'm only doing 4 masks, not 500. PIXI drops 30FPS with 4 masks on mobile. How much faster is SpriteMaskedrenderer than the PIXI one? If it's a lot faster, why doesn't PIXI use that rendering method?
-
That's good to know for directly masking textures with rectangles. Unfortunately I need to do shapes e.g circular arc masks and it needs to work on containers with multiple sprites. Can you describe what the masking process is doing in WebGL? If you set a tint value, I assume it just adds a value to every pixel. When you set a mask, can't it just copy the pixels from a mask into the alpha buffer of the masked object very quickly? What is slowing it down so much?
-
I made up a small demo scene. The problem is on mobile, if you drop it on a server somewhere and load it on any mobile device, you will see the performance issue. On desktop, it supports over 500 masked sprites no problem. On mobile, it drops 30FPS with just 4 sprites. The demo has the number set to 16 (maxSprites = 16) and the FPS drops to under 10FPS. When you turn masking off by commenting out gate.mask = gate_mask, mobile is smooth up to over 100 sprites. If there's a faster way to do masking, even if it's WebGL-only perhaps with a shader, then I could use that to maintain performance on mobile. pixi-mask.zip
-
I managed to get a slower method working but not usable in real-time for animation as it takes about 0.2-0.5 seconds to generate: - draw sprites into a rendertexture - draw masks into a rendertexture (same size as first rendertexture); - get pixel buffers for each using renderTexture.getPixels() - iterate over the buffer and draw a new PIXI Graphic using beginFill/endFill per pixel (drawRect 1x1) using color from sprite buffer and alpha from the mask buffer - convert the graphic to a texture using renderTexture.generateTexture(Graphic) This lets me manually create a static masked sprite in v3 and hundreds of sprites can run at 60 FPS. The slowest parts seem to be rendering the graphics into the buffer. What I could do for animation is render the whole mask into a larger buffer and then just read a portion of when generating the masked graphic. This would probably get it down to around 0.05-0.1 seconds per frame. This is still too high though, needs to be around 10ms (0.01s) tops . I need a faster way of converting a buffer of pixel values into a texture. Is there a low-level way of doing this like a WebGL call? Is Spine using direct WebGL calls for masking?
-
These options sound viable. I have managed to extract the pixel values from sprite and mask, how do I create a texture from a buffer of pixel values? There seems to be a BufferResource: https://github.com/db-noop/pixi.js/commit/53f98973b16710131646431385f2c82c4e5a21b9 Which Pixi version has the fromBuffer option? I can't find BufferResource to be able to create a texture from a buffer: https://pixijs.download/v5.0.0-alpha.2/docs/PIXI.resources.BufferResource.html I downloaded the source on dev branch but can't find BufferResource there. "Next" branches are all 404: https://github.com/pixijs/pixi.js/tree/dev/src
-
Pixi usually performs really well even with heavy scenes. I can put hundreds of objects, even thousands of particle sprites and get 60 FPS on mobile. I typically only use a single masked object in a scene but recently needed to use more and I was surprised at how badly Pixi performed with masked objects. For every masked sprite, I lost about 5 FPS on mobile (both Android and iOS on old and new phones, newer phones actually performed worse in some cases). By the time I added 4 masked sprites, I was down to 40 FPS, adding 10-20 masked sprites dropped to 20 FPS with really bad stuttering. As soon as I switch the mask off by removing the assignment, it goes from 20 FPS to 60 FPS, even with 200 of the same sprites. It doesn't seem like it should take so much resources to do masking given all the other effects that are possible. Tinting for example, adds a value to every pixel and costs nothing. Masking is just checking a pixel in one object and setting the equivalent pixel in the other object. Is there a faster alternative to using object.mask = mask? Is there a graphics buffer I can use to set the pixel values myself, e.g if I could create an array of pixel values and generate a texture buffer from that. Javascript is pretty fast with arrays. The main thing that bothers me is that the low performance happens with static masks. I could understand the performance hit when the sprite is animating relative to the mask but not when they are both static. Why doesn't it buffer the masked sprite and use that over and over like a normal sprite? I found a thread that describes the same issue, unfortunately I'm stuck on v3 for now: https://github.com/pixijs/pixi.js/issues/2163 I just found the following with a possible alternative using multiply blending: https://github.com/pixijs/pixi.js/issues/3735 To isolate it, it suggests using a voidfilter. Is this the fastest way to do masking in Pixi? If so, is there example code for this? Say that I did at some point want to draw a texture pixel by pixel, one way would be to draw a tinted 1x1 pixel sprite into a render texture. Is there a better way than this e.g set values in a buffer and convert it to a texture? Is there a way to read a pixel color/alpha value from a sprite or texture. There seems to be an extract function for WebGL and Canvas but it looks like this extracts the viewport. It would be good to be able to render sprites to a rendertexture and be able to read the pixel values of that texture using pixel co-ordinates.