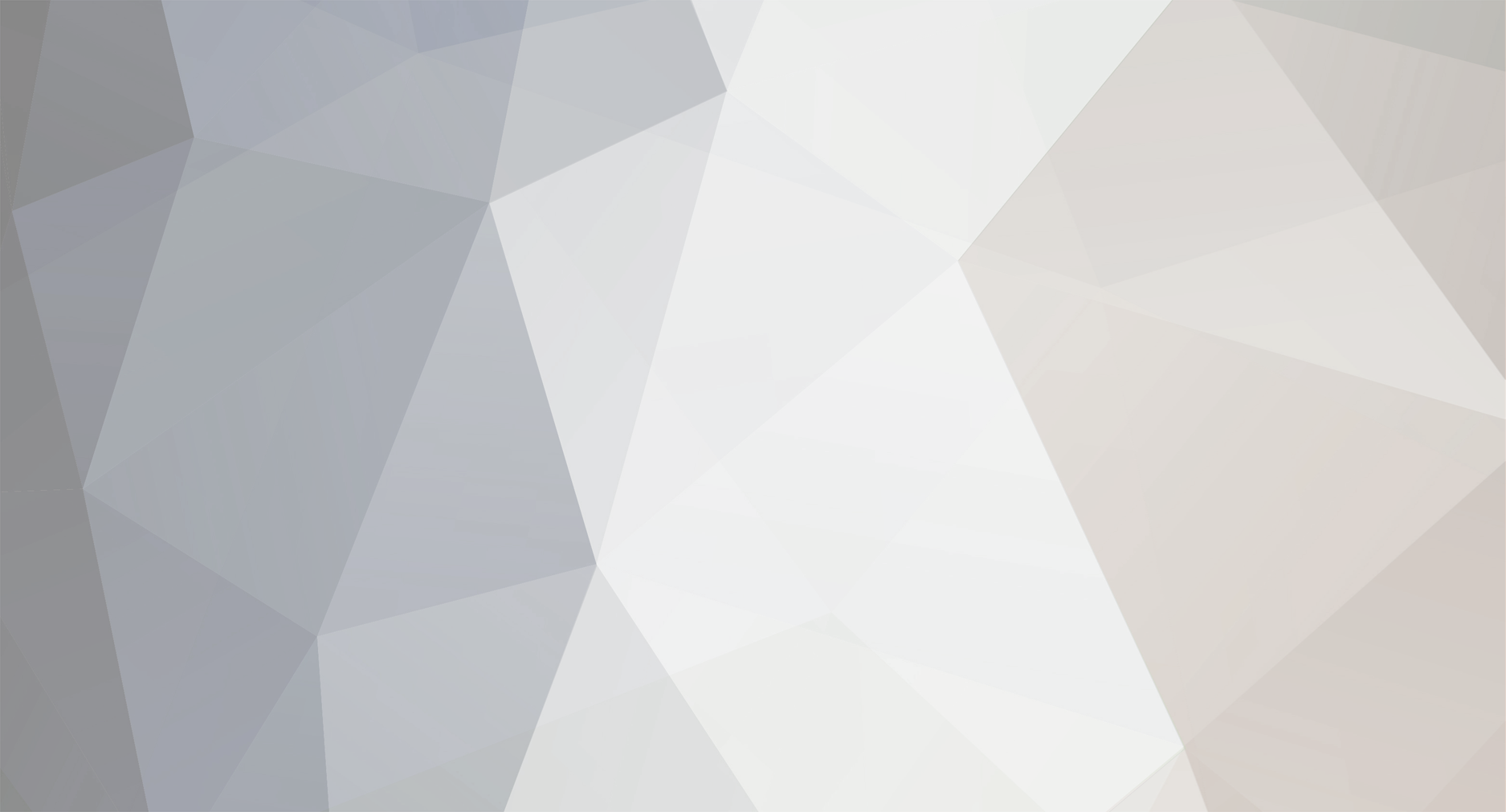
old_blueyes
Members-
Posts
13 -
Joined
-
Last visited
old_blueyes's Achievements
Newbie (1/14)
0
Reputation
-
Hi, I am trying to sort an array on a sprites x position, and display when they pass a checkpoint. Whilst it largely works: There are occasions, it will go out of sync: Can someone with a fresh pair of eyes, please look over it, i'm going round in circles: var checkPointGroup; var checkPointChild; var checkPoint; var carGroup; var car; var carQty = 2; var text; var posText1; var posText2; var obstaclesGroup; var obstaclesCollider; var obstaclesCount = 0; var obstaclesQty = 8; var obstacles; var startLine; var goTime = false; var carJump = false; class CommSc extends Phaser.Scene { constructor() { super({ key: 'CommSc' }); } preload() { this.load.json('gameConfig', 'assets/config.json'); } create() { checkPointGroup = this.physics.add.group(); carGroup = this.physics.add.group(); obstaclesGroup = this.physics.add.group(); text = this.cache.json.get('gameConfig'); posText1 = this.add.text(16, 16, '', { fontFamily: 'Arial', fontSize: '24px' }); posText2 = this.add.text(16, 42, '', { fontFamily: 'Arial', fontSize: '24px' }); // trigger startline this.timedEvent = this.time.addEvent({ delay: delayStart, callback: this.addStartLine, callbackScope: this, loop: false }); } jumpObstacles(item, obstacles) { var carID = item.texture.key.replace('racecar', ''); if(carJump) { item.body.checkCollision.none = true; item.anims.play('jump' + carID); this.time.addEvent({ delay: 2000, callback: function() { item.body.checkCollision.none = false; }, callbackScope: this, loop: false }); } carJump = true; } checkPointPass(item, checkPoint) { var carID = item.texture.key.replace('racecar', ''); if(checkPointPassed) { item.body.checkCollision.none = true; text.cars[carID].checkpoint = item.x; this.time.addEvent({ delay: 2000, callback: function() { item.body.checkCollision.none = false; }, callbackScope: this, loop: false }); } checkPointPassed = true; } displayPos() { text.cars.sort(function(a, b){return b.checkpoint - a.checkpoint}); //console.log('1st: ' + text.cars[0].name + ' XPos: ' + text.cars[0].checkpoint); //console.log('2nd: ' + text.cars[1].name + ' XPos: ' + text.cars[1].checkpoint); posText1.setText('1: ' + text.cars[0].checkpoint).setStyle({ fontFamily: 'Arial', fontSize: '24px', fill: text.cars[0].colour1 }); posText2.setText('2: ' + text.cars[1].checkpoint).setStyle({ fontFamily: 'Arial', fontSize: '24px', fill: text.cars[1].colour1 }); posText3.setText('3: ' + text.cars[2].checkpoint).setStyle({ fontFamily: 'Arial', fontSize: '24px', fill: text.cars[2].colour1 }); } addOneObstacle() { obstacles = obstaclesGroup.create(windowWidth * 2, windowHeight - 190, 'obstacles'); obstacles.setScale(0.5, 0.5); obstacles.setSize(120, 550).setOffset(80, 56); obstacles.body.velocity.x = -500; obstacles.checkWorldBounds = true; obstacles.outOfBoundsKill = true; obstaclesCollider = this.physics.add.overlap(obstaclesGroup, car, this.jumpObstacles, null, this); this.triggerChecks(); obstaclesCount++; if (obstaclesCount === obstaclesQty) { this.timedEvent.remove(false); //this.triggerFinish(); } } triggerChecks() { var fpDelay = Phaser.Math.Between(10000,22000); var cpDelay1 = (fpDelay / 4); var cpDelay2 = (fpDelay / 4) * 2; var cpDelay3 = (fpDelay / 4) * 3; this.timedEvent = this.time.addEvent({ delay: cpDelay1, callback: this.addCheckPoint, callbackScope: this }); this.timedEvent = this.time.addEvent({ delay: cpDelay2, callback: this.addCheckPoint, callbackScope: this }); this.timedEvent = this.time.addEvent({ delay: cpDelay3, callback: this.addCheckPoint, callbackScope: this }); this.timedEvent = this.time.addEvent({ delay: fpDelay, callback: this.addOneObstacle, callbackScope: this }); } addCheckPoint() { checkPoint = this.add.zone(100, 550); checkPointChild = checkPointGroup.create(windowWidth * 2, windowHeight - 190, checkPoint); this.physics.world.enable(checkPointChild); checkPointChild.setScale(0.5, 0.5); checkPointChild.setSize(100, 550); checkPointChild.body.velocity.x = -540; checkPointChild.checkWorldBounds = true; checkPointChild.outOfBoundsKill = true; this.displayPos(); this.physics.add.overlap(checkPointGroup, car, this.checkPointPass, null, this); } addStartLine() { startLine = this.physics.add.sprite(windowWidth * 2, windowHeight - 190, 'finish'); startLine.setScale(0.5, 0.5); startLine.body.velocity.x = -800; startLine.checkWorldBounds = true; startLine.outOfBoundsKill = true; this.physics.add.overlap(carGroup, startLine, this.fastForward, null, this); } carsRacing() { car = carGroup.getChildren(); for (var i = 0; i < carQty; i++) { Phaser.Actions.ScaleXY(carGroup.getChildren(), -0.035, -0.035, -0.002, -0.002); car[i].depth += car[i].y; car[i].setX(Phaser.Math.Between(0, 200)); car[i].setSize(265, 210, true).setOffset(50, 80); } } fastForward(item, startLine) { goTime = true; if (!startLine.hasOverlapped && !item.hasOverlapped) { startLine.hasOverlapped = item.hasOverlapped = true; this.triggerChecks(); } } } Any help is hugely appreciated
-
Hi, I'm after some advice, i'm trying to figure out the most efficient way of emulating the behaviour of the commentary bar within Football Manager series. In essence a notification bar, which shows up intermittently and displays text highlights: Now, i've already done a little groundwork and have a renderBox() function that when called, will display bar and message, then disappear. I'm wondering though what is the most efficient way of the achieving random intermitten display behaviour? Would anybody recommend a setTimeout approach, I have also wondered about firing it on boolean triggers. Appreciate any feedback. Thanks
-
Thanks, just tried it and it worked ? Strangely though, i'm sure I tried this last night and it didn't work. ?
-
old_blueyes reacted to a post in a topic: destroy group
-
Hi, Having problems using .destroy() to remove a group. Am i using it wrong? Is there an alternative I should use? I've tried .kill() and .remove() and they don't work either, my code is below. Destroy is at the very bottom in the hideCommentaryBox() function. preload() { } create() { this.timedEvent = this.time.addEvent({ delay: 1500, callback: this.singleLineCommentary, callbackScope: this}); } update() { } singleLineCommentary() { this.showCommentaryBox("HELLO THERE! Put Some Text Here!"); this.timedEvent = this.time.addEvent({ delay: 4500, callback: this.hideCommentaryBox, callbackScope: this}); } showCommentaryBox(text) { if (this.commBox) { this.commBox.destroy(); } var commBox = this.add.group(); var rect = new Phaser.Geom.Rectangle(0, (960*scaleFactor), windowWidth, 70); var commBack = this.add.graphics({ fillStyle: { color: 0x0000ff } }); commBack.fillRectShape(rect); rect.depth += windowHeight; var style = { fontSize: 24, fontFamily: 'Arial', align: "center" } var commText = this.add.text(windowWidth / 2, (1020*scaleFactor), text, style).setOrigin(0.5, 0.5); commBox.add(commBack); commBox.add(commText); this.commBox = commBox; } hideCommentaryBox() { console.log('hide'); this.commBox.destroy(); }
-
many thanks both, hugely appreciated... also some good advice
-
Sorry to bump the post, but could anyone please help?
-
Hi, Hope somebody can help, I have a sprite which i've coded to animate on overlap. But it's acting very strangely, currently when the sprite overlaps it will trigger the first frame of the animation. Which stays as a still frame, until the sprite finishes the overlap of which then the rest of the animation plays out. It's like there's a delay on the animation somewhere, can't figure out where. If there is, it would be strange as it switches to the first frame of the animation. But it should be one continuous motion. Appreciate it if anyone can take a look: https://codepen.io/old_blueyes/pen/GXxBmm Thanks
-
Hi All, Making a racing game, very much a work in progress. I am struggling however, with the in-race action. I am trying to use random velocity on the individual runners, for them to move up and down the field. Whilst it works from time-to-time, a lot of the time though the runners get stuck together and move up and down as one group, which is not what I want. I am hoping for the in race action to be more organic, less bunched together. https://codepen.io/old_blueyes/project/editor/DQyeLQ#0 Does anyone have any suggestions?? Thanks
-
Hi, I am making a landscape game, just curious about dimensions. I understand, there maybe dependencies on image sizes etc, but are there a set of default dimensions to aim for?? What does everyone else use? Thanks
-
Hi, Is it possible to run 2 separate timelines one after another? i.e. using the onComplete parameter on the first timeline to fire a function which contains the second timeline. using something similar to this: var timeline = this.tweens.timeline({ tweens: [{ targets: targs, x: startPos, duration: startDur, ease: 'Sine.easeInOut', delay: delay }, { targets: targs, x: jostlePos, duration: lineDur, ease: 'Sine.easeInOut', yoyo: true, repeat: 2, onComplete: function () { var timeline2 = this.tweens.timeline({ tweens: [{ targets: targs, x: 1000, duration: raceDur, ease: 'Sine.easeInOut', delay: delay }] }); console.log('complete'); } }] }); All i get is Cannot read property 'timeline' of undefined, which is referring to the second timeline, as the first plays without bother
-
Anyone? Please am willing to make a donation for your efforts.
-
Hi, I hope that I find you well, I am a little stuck. I have a group of sprites, that on overlap of another jump sprite image, I require it to play the jump animation, but only on the individual sprite within the group, when it has overlapped. My code, is pretty much working how I want apart from this issue, in the current build, on overlap the animation will play for all sprites within the group (not an individual basis), and will trigger again (beyond the original, overlap) if another sprite hits the jump image. Appreciate any assistance. var game; var globalParams = { calculatedWidth: 1350, calculatedHeight: 900 } var GameResolution = { _1350: 1350, _960: 960 }; function calculateSize() { // Get Pixel Width and Height of the available space var w_w = window.innerWidth * window.devicePixelRatio; var w_h = window.innerHeight * window.devicePixelRatio; var g_w = w_w; // We prepared all assets according to aspect ratio of 1.5 // Since we are going to use different assets for different resolutions, we are not looking at the resolution here var originalAspectRatio = 1.5; // Get the actual device aspect ratio var currentAspectRatio = g_w / w_h; if (currentAspectRatio > originalAspectRatio) { // If actual aspect ratio is greater than the game aspect ratio, then we have horizontal padding // In order to get the correct resolution of the asset we need to look at the height here // We planned for 1350x900 and 960x640 so if height is greater than 640 we pick higher resolution else lower resolution if(w_h > 640){ globalParams.selectedResolution = GameResolution._1350; globalParams.calculatedHeight = 900; globalParams.calculatedWidth = w_w * (900 / w_h); } else { globalParams.selectedResolution = GameResolution._960; globalParams.calculatedHeight = 640; globalParams.calculatedWidth = w_w * (640 / w_h); } } else { // If actual aspect ratio is less than the game aspect ratio, then we have vertical padding // In order to get the correct resolution of the asset we need to look at the width here if(w_w > 960){ globalParams.selectedResolution = GameResolution._1350; globalParams.calculatedWidth = 1350; globalParams.calculatedHeight = w_h * (1350 / w_w); } else { globalParams.selectedResolution = GameResolution._960; globalParams.calculatedWidth = 960; globalParams.calculatedHeight = w_h * (960 / w_w); } } } window.onload = function () { calculateSize(); game = new Phaser.Game(globalParams.calculatedWidth, globalParams.calculatedHeight, Phaser.AUTO, 'gameDiv'); game.state.add('main', mainState); game.state.start('main'); //game.forceSingleUpdate = true; } var yourGroup; var mainState = { preload: function() { if(!game.device.desktop) { game.scale.scaleMode = Phaser.ScaleManager.SHOW_ALL; game.scale.setMinMax(game.width/2, game.height/2, game.width, game.height); } game.scale.pageAlignHorizontally = true; game.scale.pageAlignVertically = true; // Background game.stage.backgroundColor = '#71c5cf'; // Horse Sprites for (var i = 1; i <= 3; i++) { this.load.spritesheet('runner'+i, 'assets/horse-'+i+'.png', 368, 300); } // Other Sprites game.load.image('fence', 'assets/jumpfence1.png'); game.load.image('track', 'assets/grasstrack1.png'); }, create: function() { this.jumpGroup = game.add.group(); this.timer = game.time.events.loop(12000, this.addNewJump, this); this.horseGroup = game.add.group(); this.horseGroup.enableBody = true; this.horseGroup.physicsBodyType = Phaser.Physics.ARCADE; this.addHorse(this); }, addHorse: function() { for (var i = 1; i <= 3; i++) { var posY = game.rnd.integerInRange(300, 500); var posX = game.rnd.integerInRange(30, 120); geegee = this.horseGroup.create(posX, posY, 'runner'+i); // Add 'sprite' to the group this.horseGroup.sort('y', Phaser.Group.SORT_ASCENDING); geegee.animations.add('gallop', [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 20, true); geegee.animations.add('jump', [4, 11, 12, 13, 14, 15, 16, 17, 4], 14, false); } }, addJump: function(x, y) { var fence = game.add.sprite(x, y, 'fence'); this.jumpGroup.add(fence); game.physics.arcade.enable(fence); fence.body.setSize(50, 350, 105, 0); fence.body.velocity.x = -500; fence.checkWorldBounds = true; fence.outOfBoundsKill = true; }, addNewJump: function() { this.addJump(game.world.width - 40, this.game.height - this.game.cache.getImage('fence').height); }, update: function() { this.horseGroup.forEach(function(item) { // jump fences this.game.physics.arcade.overlap(this.horseGroup, this.jumpGroup, this.fenceJump, null, this); this.hurdle(); // move up and down field if (item.x > game.width - 650) { item.x = game.width - 650; item.body.velocity.x += game.rnd.integerInRange(-5, 0); } else { item.body.velocity.x += game.rnd.integerInRange(-5, 5); } if (item.x < 50) { item.x = 50; item.body.velocity.x = 0; } }.bind(this)); }, fenceJump: function(horseGroup,jumpGroup) { this.horseGroup.forEach(function(item) { item.body.velocity.y = 1; hurdleFence=false; }.bind(this)); }, hurdle: function() { this.horseGroup.forEach(function(item) { if(!this.hurdleFence){ //check if we already have -50 gravity or not if(item.body.velocity.y > 0){ item.events.onAnimationStart.add(airJump, this); function airJump() { item.body.velocity.x = 5; item.body.gravity.x = 100; } item.animations.play('jump'); item.events.onAnimationComplete.add(groundGallop, this); function groundGallop() { item.body.velocity.y = 0; } } else { item.animations.play('gallop'); } } }.bind(this)); }, };