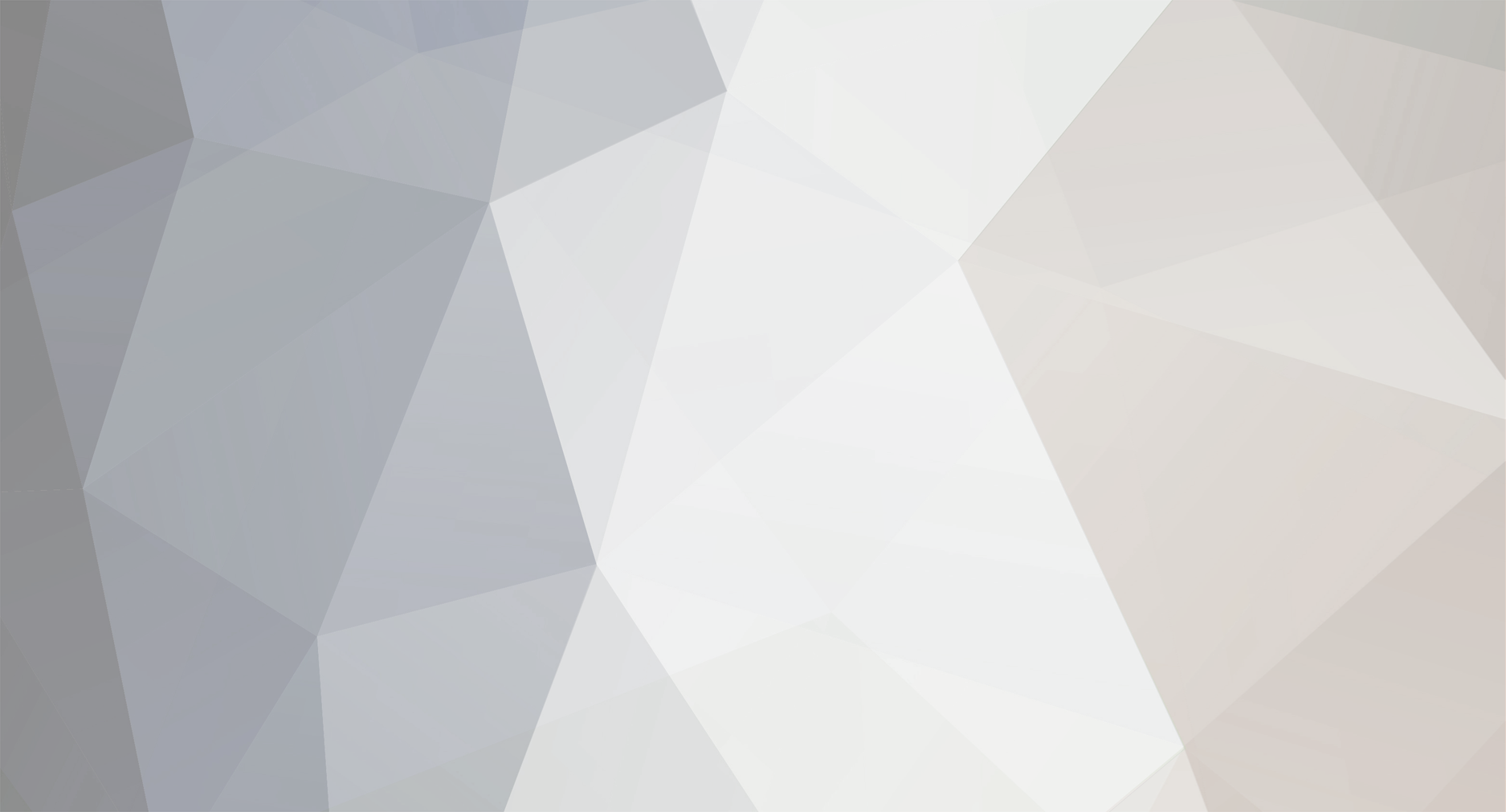
Jambutters
Members-
Posts
52 -
Joined
-
Last visited
Everything posted by Jambutters
-
Made a feature request : https://github.com/photonstorm/phaser/issues/4090 // //create shared animations createAnimations([player, monster, npc], "right", [0,1,2,3]) createAnimations([player, monster, npc], "up", [0,1,2,3]) createAnimations([player, monster, npc], "down", [0,1,2,3]) createAnimations([player, monster, npc], "left", [0,1,2,3]) function createAnimations(texture, animationName, frames) { for (var i = 0; i < texture.length; i++) { this.anims.create({ key: texture[i] + animationName, frameRate: 5, frames: this.anims.generateFrameNumbers(texture, { frames: frames }) }); } } //sprites have a .textureKey property and when they play animation, sprite.anims.play(this.textureKey+animationName); But yeah, duplicating objects, I wonder how it's going to impact performance.
-
Ahh, my bad, not used to webpack.
- 10 replies
-
The example folder isn't bundled to be ran.
- 10 replies
-
Think melonjs has a feature like that. Provide a test case!
-
Duplicate frames in SpriteSheet removal
Jambutters replied to Jambutters's topic in Coding and Game Design
Nevermind, Texturepacker has the feature -
So I have a lot of spritesheets (32x32) that have the exact same frame of sprite, and I want to remove the duplicates. Does imagemagick have a function to scan images pixel by pixel and delete any duplicates? That or is there a program that can detect duplicate frames? Going to need to apply this for 100s of spritesheets.
-
How many of you guys use delta in your game loops ? I've adapted my game loop to this: https://isaacsukin.com/news/2015/01/detailed-explanation-javascript-game-loops-and-timing but the game I'm working on has no sense of gravity, and is a tile/grid based movement game, tl;dr everything can only move 32px. What are the pros and cons of using delta? Do you use delta?
-
Currently using Tiled and my maps have multiple layers : Something like: //Floor: let floor = [0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0] //Trees: just pretend it's filled with different values let trees = [0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0] So how I'm going about it is to generate a PIXI.Sprite for every tile and then pushing those into a container, and that container would be the layer: //an array of PIXI.Textures sliced from the spritesheet for tiles let tileSpriteResources = [texture, texture, texture]; let floorLayer = new PIXI.Container(); let trees = new PIXI.Container(); //something like this: function makeLayerSprites(containerLayer,array, width, height){ let W = width; let D = height; let shiftY = 0; array.map(function(item, index){ if(index >= W){ //shifts Y downwards when X is passed with width shiftY++; } if(item === 0){ let tile = new PIXI.Sprite(tileSpriteResources[0]); tile.setTransform(index * 32, shiftY * 32); containerLayer.addChild(tile); } }); } I'm going to have like.. 6+ layers and I was wondering if I should just generate 1 layer, and then have the rest of them be the children of every PIXI.Sprite on the first layer. Or is my approach just fine ? For culling I'd imagine I would have to loop through all 6 containers children and yeah, I'm not sure how this will strike me in performance. Any general advice or recommendations or how you've implemented multi-layered maps in PIXI ?
-
let app = new PIXI.Application({ autoStart: true, width: 640, height: 480 }); PIXI.loader.resoures.add("./spritesheet.json"); /* creates a Texture using the ENTIRE spirtesheet image instead of reading from json and cropping it to texture atlas */ let testTexture = new PIXI.Texture(PIXI.loader["./spritesheet.json"].textures["player2.png"]); //work around : new PIXI.Texture(PIXI.loader["./spritesheet.json"].textures["player2.png"],PIXI.loader["./spritesheet.json"].textures["player2.png"].orig); or by using .frame /* What's the point of passing ["player2.png"], specifying the texture atlas location if it's just going to use the entire image when creating calling new PIXI.Texture . */ let testSprite = new PIXI.Sprite(testTexture); /* this corrects the sprite with the correct frames though referencing the texture in the loader*/ let testTexture2 = PIXI.loader["./spritesheet.json"].textures["player2.png"].texture; let testSprite2 = new PIXI.Sprite(testTexture2); //correct crop refferencing texture atlas frame. I know how to work around this but shouldn't "new PIXI.Texture" reach into the json file and grab the correct location of the image rather than use the entire image of the spritesheet?
-
Found the problem... Unsure if this is a bug. I am grabbing the correct texture which is 200px down but it's not using the grabbed texture starting location @ Y: 200px down , as it's starting point for x: 0, y: 0, rather it's always starting from the entire sprite sheet... Can fix from reading the json file though. Is there another way to deal with this ? Resolvedish ..
-
Bad effects in the texture constructor? I am debugging with console.logs everywhere and can't seem to find the problem but I'll try w/ debugger and see if I can catch anything. Really strange problem...I am passing the correct string though... Does the first object in the atlas get referenced first if there is an error or if the fail to specify what image you want in the atlas? . Is there a way to check what image I'm representing when creating a texture using ``` new PIXI.Texture(PIXI.loader.resources['atlas.json'].textures["theimage.png"]) ``` ? Like, yeah, is there a way to check "theimage.png" value referenced on the texture object? Narrowed down the problem a bit more. It works and references correct sprite fine when creating a PIXI.Sprite , but when doing a PIXI.extras.AnimatedSprite, it always gets it wrong it seems?
-
Also how would you check if a texture exists in the atlas or not? Trying this: if (loaderSource[atlasJson].textures[srcImg]) { //logic } and it gives me Uncaught TypeError: Cannot read property 'textures' of undefined. edit: nevermind , fixed this type error
-
does the "let" scope to the block ? Changed it and no luck :s.
-
I'm pretty new to JS so I apologize for the messy code. Also don't hold back on telling me what I should and shouldn't do design wise! The problem is that way at the bottom where the for loop is, it is not referencing the textures I want as declared in the variables head and body early on. But when I test it out in about line 16 or so, it does reference the correct texture.Thanks for reading class Player { constructor(dataObj) { // this.uid = dataObj.username; this.sprite = dataObj.sprite || "default"; this.x = dataObj.x || 0; this.y = dataObj.y || 0; this.width = 32; this.height = 32; this.PIXISPRITE; this.testSprite; if (dataObj.sprite.custom === false) { let spriteTextures = []; let head = loaderSource["/client/img/custom_head.json"].textures[this.sprite.layer1 + ".png"]; let body = loaderSource["/client/img/custom_body.json"].textures[this.sprite.layer2 + ".png"]; spriteTextures.push(head, body); console.log(head); this.testSprite = new PIXI.Sprite(head); /*when I test this, it grabs the texture from atlas just fine. */ let createArrComponents = (paraSpriteText) => { let arrayTextures = []; let counterX = 0; let counterY = 0; for (let i = 0; i < paraSpriteText.length; i++) { let tmpArray = []; let indexspriteTextures = i; const ROWS = paraSpriteText[i].width / this.width; const COLUMS = paraSpriteText[i].height / this.height; for (let i = 0; i < ROWS * COLUMS; i++) { let rectFrameObj = new PIXI.Rectangle(counterX, counterY, this.width, this.height); counterX += this.width; let framedTexture = new PIXI.Texture(paraSpriteText[indexspriteTextures]); framedTexture.frame = rectFrameObj; tmpArray.push(framedTexture); if (counterX >= this.width * ROWS) { counterX = 0; counterY += this.height; } } arrayTextures.push(tmpArray); } return arrayTextures; }; let spriteComponentsArr = createArrComponents(spriteTextures); console.log(spriteComponentsArr); for (let i = 0; i < spriteComponentsArr.length; i++) { let headSprite = new PIXI.extras.AnimatedSprite(spriteComponentsArr[0]); /*THESE TWO ARE NOT REFFERENCING THE TEXTURE I WANT AS DECLARED IN THE VARIABLES head & body */ this.PIXISPRITE = headSprite; headSprite.addChild(new PIXI.extras.AnimatedSprite(spriteComponentsArr[1])); //ADDSSTHEBODY } } Player.list[this.uid] = this; } createSelfSprite() { } } let test = new Player({username: "testy", sprite: {layer1: "head_no1", layer2: "body_no6"} });
-
OOHHH. I see. Using typescript and if the first parameter for the Texture class is not a base texture, I get a warning so I never bothered to try it.
-
What are the things that differentiate the two ? Example: let texture = PIXI.loader.resources("player.png).texture; let otherTexture = new PIXI.Texture(PIXI.loader.resources("player.png).texture); I assumed they were the same thing and I don't understand the mechanisms behind the two.
-
Actually never mind... forgot that let croped does not create a copy , rather points directly to the texture
-
let loaderSource = PIXI.loader.resources; let initPlayer = ():void =>{ let croped = PIXI.loader.resources["./img/player.png"].texture; let t32Rect = new PIXI.Rectangle(0, 0, 32, 32); // console.log(croped); croped.frame = t32Rect; let player = new PIXI.Sprite(loaderSource["./img/player.png"].texture); //this is not displaying the entire image, rather it's using the .frame clip. Shouldn't this be the independent whole image/texture? rootStage.addChild(player); console.log(player); }; Not sure why the Sprite displayed on PIXI is a cropped image rather than the whole thing. Shouldn't it be the entire thing ?
-
Noob to game dev here, this is more of a general javascript question but: I'm looking over kittykatattacks spriteUtilities library and var frameRate = 1000 / sprite.fps; //Set the sprite to the starting frame sprite.gotoAndStop(startFrame); //Set the `frameCounter` to the first frame frameCounter = 1; //If the state isn't already `playing`, start it if (!sprite.animating) { timerInterval = setInterval(advanceFrame.bind(this), frameRate); } So how does this(the sprites fps) not conflict with the games fps? Generally you have the main game update loop somewhere that's also a setInterval(gameLoop, fps). and javascript is synchronous right?
-
Thanks mate! Indeed I do need a lot of practice, hopefully I at least become proficent in 3-4 months. I've been reading a lot of resources on js over and over but it does not stay in my head if I do not use it lol. Anyways, what do you mean by removing the "=" in the for loop? Solved the problem by issuing a break statement in the if statement opposed to returnning undefined P: . (wtf, though, thought return undefined; takes me out of the for loop only, not the entire function).
-
So I'm trying to splice all frames from an image, convert them to textures then put them into an array but I'm not sure as to why it is erroring, also I am also not sure why the if statements need to be above the "let rect" declarations let createArrayTexture = function(source, frameWidth, frameHeight){ let textureArray = []; let frameX = 0; let frameY = 0; let totalFrames = PIXI.loader.resources[source].texture.width/32 * PIXI.loader.resources[source].texture.height/32; for(let i = 0; i <= totalFrames; i++){ console.log(`${i}, frameX: ${frameX} frameY: ${frameY}`); let tempTexture = new PIXI.Texture(PIXI.utils.TextureCache[source]); if(frameX === PIXI.loader.resources[source].texture.width){ frameX = 0; frameY+=32; } if(frameY === PIXI.loader.resources[source].texture.height){ return; } let rect = new PIXI.Rectangle(frameX, frameY, frameWidth, frameHeight); tempTexture.frame = rect; textureArray.push(tempTexture); frameX+=32; //console.log(tempTexture); } return textureArray; }; let createPlayer = function(){ let animationArray = createArrayTexture("./img/player.png", 32, 32); let frameFlipChar = new PIXI.extras.AnimatedSprite(animationArray); }; Solution: Thought return undefined would only break out of for loop, not entire function
-
Alright, found the problem, its with setInterval, but I want to be able to use it opposed to requestframeanimation because I want to control the fps... unsure how to tackle the problem
-
Probably not a problem with Pixi(most likely I'm just using pixi wrong and somewhere leaked) but somewhere there is a memory leak and I am not sure why. Ram usage keeps increasing and movement is very laggy even when I set to 5 from 30fps. If anyone can figure out why, thanks :D. (I am very beginner js, if you have good resources on debugging please link) or is it just my computer... ? (Also noted... the image rendered is the entire sprite sheet because I need to mess with player.frame more to add animation). Using nodejs to host webserver (function(){ let renderer = new PIXI.WebGLRenderer(640,480); document.body.appendChild(renderer.view); let rootStage = new PIXI.Container(); let Unit = function(img, hp, type, spdX, spdY){ let entity = new PIXI.Sprite(PIXI.loader.resources[img].texture); entity.img = img; entity.hp = hp; entity.type = type; entity.spdX = spdX; entity.spdY = spdY; entity.pressingDown = false; entity.pressingUp = false; entity.pressingLeft = false; entity.pressingRight = false; return entity; }; let player; let createPlayer = function(){ let tmpplayer = Unit("./img/player.png", 150, "player", 0.1, 0.1); //msp should be 16 but for some reason it moves me out of the map.. why is it so high/fast?? player = tmpplayer; rootStage.addChild(tmpplayer); }; let setup = () => { createPlayer(); updateLoop(); }; document.onkeydown = function(event){ if(event.keyCode === 87){ //w player.pressingUp = true; }else if(event.keyCode === 65){ //a player.pressingLeft = true; }else if(event.keyCode === 83){ //s player.pressingDown = true; }else if(event.keyCode === 68){ //d player.pressingRight = true; } }; document.onkeyup = function(event){ if(event.keyCode === 87){ //w player.pressingUp = false; }else if(event.keyCode === 65){ //a player.pressingLeft = false; }else if(event.keyCode === 83){ //s player.pressingDown = false; }else if(event.keyCode === 68){ //d player.pressingRight = false; } }; let updatePlayerPos = function(){ if(player.pressingUp === true){ player.y -= player.spdY; }if(player.pressingLeft === true){ player.x -= player.spdX; }if(player.pressingDown === true){ player.y += player.spdY; }if(player.pressingRight === true){ player.x += player.spdX; } }; let updateLoop = function(){ updatePlayerPos(); renderer.render(rootStage); setInterval(updateLoop, 1000/25); }; PIXI.loader.add("./img/player.png").load(setup); }());
-
let Unit = function(img, hp, type, spdX, spdY){ let entity = new PIXI.Sprite(PIXI.loader.resources[img].texture); entity.img = img; entity.hp = hp; entity.type = type; entity.spdX = spdX; entity.spdY = spdY; rootStage.addChild(entity); return entity; }; let setup = () => { let player = Unit("./img/player.png",150, player); //error , player is undefined. console.log(player); updateLoop(); }; I get an error of player is undefined as you can see but I'm not exactly sure as to why. This is more of an understanding javascript question, sorry.
-
Open source SpriteSheet Packer with GUI
Jambutters replied to amakaseev's topic in Coding and Game Design
Sweet, thanks for making an alternative to the texturepacker monopoly. SAVE US